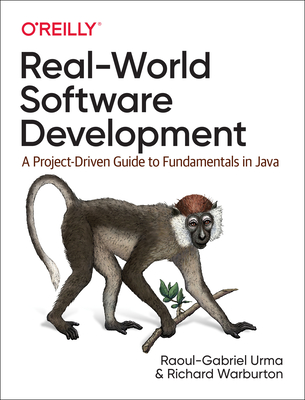
Real-World Software Development: A Project-Driven Guide to Fundamentals in Java
內容描述
From the Preface
Mastering software development involves learning a disparate set of concepts. If you’re starting out as a junior software developer, or even if you’re more experienced, it can seem like an insurmountable hurdle. Should you be spending time learning about established topics in the object-oriented world such as SOLID principles, design patterns, or test-driven development? Should you be trying out things that are becoming increasingly popular such as functional programming?
Even once you’ve picked some topics to learn it’s often hard to identify how they fit together. When you should go down the route of applying functional programming ideas in your project? When do you worry about testing? How do you know at what point to introduce or refine these techniques? Do you need to read a book on each of these topics and then another set of blog posts or videos to explain how to put things together? Where do you even start? Don’t worry, this book is here to help you.
You'll be helped through an integrated, project-driven approach to learning. You’ll learn the core topics you need to know in order to become a productive developer. Not only that, but we show how these things fit together into bigger projects.
Each chapter is structured around a software project. At the end of a chapter, if you’ve been following along, you should be able to write that project.
The projects start off as simple command-line batch programs, but grow in complexity to fully fledged applications.
A Developer-Oriented Approach
This book also gives you the opportunity to learn in a developer-oriented way. It contains plenty of code samples and whenever we introduce a topic we always provide concrete code examples.
We cover all the SOLID principles throughout various chapters. These are a set of principles designed to help make software easier to maintain.
Single Responsibility Principle (SRP), discussed in Chapter 2
Open/Closed Principle (OCP), discussed in Chapter 3
Liskov Substitution Principle (LSP), discussed in Chapter 4
Interface Segregation Principle (ISP), discussed in Chapter 5
Dependency Inversion Principle (DIP), discussed in Chapter 7
You get all the code for the projects within the book, so if you want to follow along you can even step through the book code in an Integrated Development Environment (IDE) or run the programs in order to try them out.
Another common bugbear when it comes to technical books is that they are often written in a formal, lecturing style. That’s not how normal people speak to each other! In this book you’ll get a conversational style that helps to engage you in the content rather than being patronizing.
Who Should Read This Book?
We’re confident that developers from a wide variety of backgrounds will find things that are useful and interesting in this book. Having said that, there are some people who will get the maximum value out of this book.
Junior software developers, often just out of university or a couple of years into their programming career, are who we think of as the core audience for this book.
You’ll learn about fundamental topics that we expect to be of relevance throughout your software development career. You don’t need to have a university degree by any means, but you do need to know the basics of programming in order to make the best use of this book. We won’t explain what an if statement or a loop is, for example.
You don’t need to know much about object-oriented or functional programming in order to get started. In Chapter 2, we make no assumptions beyond that you know what a class is and can use collections with generics. We take it right from the basics.
Another group who will find this book of particular interest is developers learning Java while coming from another programming language, such as C#, C++, or Python. This book helps you quickly get up to speed with the language constructs and also the principles, practices, and idioms that are important to write good Java code.
If you’re a more experienced Java developer, you may want to skip Chapter 2 in order to avoid repeating basic material that you already know, but Chapter 3 onward will be full of concepts and approaches that will be of benefit to many developers.
We’ve found that learning can be one of the most fun parts of software development and hope that you’ll find that as well when reading this book. We hope you have fun on this journey.
作者介紹
Dr. Raoul-Gabriel Urma is the CEO and founder of Cambridge Spark, a leader in transformational data science and AI training, career development, and progression. He is author of several programming books, including the best seller Modern Java in Action (Manning). Raoul-Gabriel holds a PhD in Computer Science from Cambridge University as well as an MEng in Computer Science from Imperial College London and graduated with first-class honors, having won several prizes for technical innovation. His research interests lie in the area of programming languages, compilers, source code analysis, machine learning, and education. He was nominated an Oracle Java Champion in 2017. He is also an experienced international speaker, having delivered talks covering Java, Python, Artificial Intelligence, and Business. Raoul has advised and worked for several organizations on large-scale software engineering projects including at Google, Oracle, eBay, and Goldman Sachs.
Richard Warburton is an empirical technologist and solver of deep-dive technical problems. Recently he has worked on data analytics for high performance computing and authored Java 8 Lambdas for O'Reilly. He is a leader in the London Java Community and organized the Adopt-a-JSR programs for Lambdas and Date and Time in Java 8. Richard also frequently speaks at conferences, and has presented at JavaOne, DevoxxUK, Geecon, Jfokus and JAX London. He obtained a PhD in Computer Science from The University of Warwick, where his research focused on compiler theory.